# MSX-2 SCREEN Concept
The **MSX-2 SCREEN concept** revolves around *multiple screen modes*, each tailored for different use cases like text, tile-based graphics, bitmapped images, and sprites. MSX-2 greatly expanded upon the original MSX video capabilities by leveraging the **Yamaha V9938** video display processor (VDP), allowing for higher resolution, more colors, and multiple VRAM pages.
---
## π¨ Overview of SCREEN Modes in MSX-2
| Mode | Type | Resolution | Colors | Description |
|------------|----------|----------------|------------------|----------------------------------------------|
| SCREEN 0 | Text | 40Γ24 (text) | 2 colors | Default text screen (40 columns) |
| SCREEN 1 | Text | 32Γ24 (text) | 15 colors (limited) | Colorful, 32-column text mode |
| SCREEN 2 | Tile | 256Γ192 | 16 (per 8Γ1 area) | Tile-based mode for games |
| SCREEN 4 | Tile | 256Γ212 | 16 (fixed palette) | MSX2 version of SCREEN 2 |
| SCREEN 5 | Bitmap | 256Γ212 | 16 (from 512) | Direct pixel graphics |
| SCREEN 6 | Bitmap | 512Γ212 | 4 (from 512) | High-res graphics mode |
| SCREEN 7 | Bitmap | 512Γ212 | 16 (from 512) | High-res, 16-color mode |
| SCREEN 8 | Bitmap | 256Γ212 | 256 (fixed) | 8-bit color mode (no sprite support) |
| SCREEN 10 | YJK | 256Γ212 | ~12,000 (YJK) | Photo-realistic color encoding |
| SCREEN 11 | YJK+PAL | 256Γ212 | ~12,000 + 16 | YJK with palette override |
| SCREEN 12 | YJK | 512Γ212 | ~12,000 | Hi-res YJK mode (MSX2+) |
---
## π¦ Concepts Behind the SCREEN System
### 1. Tile Modes vs Bitmap Modes
- **Tile-based (SCREEN 1β4):**
- Use **character patterns** and **name tables**.
- Efficient and fastβperfect for retro-style games.
- **Bitmap modes (SCREEN 5β8, 10β12):**
- Allow **per-pixel** control.
- Best for drawing, images, or artistic apps.
---
### 2. Color System
- MSX-1: fixed 16-color palette.
- MSX-2:
- **512-color palette**
- Custom palette via `COLOR=` or `VDP` register.
- Bitmap screens can use these with up to 16 or 256 colors.
- YJK modes allow 12,000+ virtual colors.
---
### 3. Pages
- Most modes support **multiple VRAM pages**.
- You can:
- Draw on one page.
- Show another page.
- Swap for **smooth animation** (double-buffering).
---
### 4. Sprites
- Used in SCREEN 1β7.
- **32 hardware sprites**, up to 16 colors.
- Limit: **4 sprites per scanline**.
---
### 5. VRAM Layout
- Use `VPOKE`, `VPEEK`, and `COPY` to directly manage VRAM:
- Tiles, bitmaps, sprite patterns, palettes, etc.
---
## π§ͺ Example: Draw a Pixel in SCREEN 5
```basic
SCREEN 5
PSET (100, 100), 15 ' White pixel
<#
.SYNOPSIS
Processes a text file by adding line numbers and replacing label-based GOTO/GOSUB commands.
.DESCRIPTION
- Each line is numbered sequentially starting from a given Start, with an increment of Step.
- Labels (lines starting with ':') are replaced with a numbered line containing "REM :label".
- GOSUB and GOTO commands referencing a label (e.g., "gosub myLabel") are replaced with the numeric line.
- Case-insensitive matching for labels and commands.
.PARAMETER FilePath
Path to the file to process.
.PARAMETER Start
Starting number for line numbering (default: 10).
.PARAMETER Step
Increment step for line numbering (default: 10).
.EXAMPLE
.RenumberWithLabels.ps1 -FilePath "C:codescript.bas" -Start 10 -Step 10
#>
param(
[string]$FilePath,
[int]$Start = 10,
[int]$Step = 10
)
if (-not (Test-Path $FilePath)) {
Write-Error "File not found: $FilePath"
exit 1
}
$lines = Get-Content $FilePath
$output = @()
$labelMap = @{}
$lineNumber = $Start
# First pass: assign line numbers and store label mappings
foreach ($line in $lines) {
$trimmed = $line.Trim()
if ($trimmed -match '^:([a-zA-Z_][w]*)$') {
$label = $matches[1]
$labelMap[$label.ToLower()] = $lineNumber
$output += "$lineNumber REM :$label"
}
else {
$output += "$lineNumber $line"
}
$lineNumber += $Step
}
# Second pass: replace GOSUB/GOTO label references
for ($i = 0; $i -lt $output.Count; $i++) {
$line = $output[$i]
foreach ($label in $labelMap.Keys) {
$line = $line -replace "(?i)bgosubs+:?$labelb", "gosub $($labelMap[$label])"
$line = $line -replace "(?i)bgotos+:?$labelb", "GOTO $($labelMap[$label])"
}
$output[$i] = $line
}
# Save to file or print
$output | Set-Content "$FilePath.processed"
Write-Host "Processed file saved as: $FilePath.processed"
Friday, April 11, 2025
MSX-2 Disk Deployment Script Requirements
This document outlines the complete functionality for a PowerShell script that builds a bootable MSX-2 .dsk image from a BASIC project.
Input Parameters
- MainBasPath (Required, String): Full path to the main .BAS file.
- ProjectName (Optional, String): Optional project name. Defaults to the base name of the .BAS file.
Folder and File Structure
Work Folder
- Location: Sibling to the folder containing MainBasPath.
- Name Format: ProjectName_Hash (the hash is 6 characters from a GUID).
- Contents: Renumbered .BAS files and AUTOEXEC.BAS.
Disk Image
- Name: ProjectName.dsk.
- Location: Same parent folder as the folder containing MainBasPath.
- Base: Created by copying the "New 720KB Boot Disk.dsk" template.
- Overwrite: If the file already exists, it is overwritten.
Steps
-
Resolve and Print All Paths
- Compute all paths (folders, tools, and output) at the top of the script and print them.
-
Create Work Folder
- Create a work folder as a sibling to the project folder using the format: ProjectName_Hash.
-
Create AUTOEXEC.BAS
- Create AUTOEXEC.BAS in the work folder.
- The file content should be: RUN "MainFile.BAS" (using the actual main file name), written in ASCII.
-
Copy and Renumber .BAS Files
- Copy all .BAS files from the folder containing MainBasPath to the work folder.
- Renumber each .BAS file by calling the external renumbering script:
D:WorkspaceMSXtoolsrenumber.ps1 -FilePath -Step 10 -Start 10
-
Create and Populate Disk Image
- Copy the template image "New 720KB Boot Disk.dsk" to the destination DiskImage path, overwriting any existing file.
- Add each file from the work folder to the disk image using the command:
dsktool.exe A "C:pathtoimage.dsk" "C:pathtofile.ext"
- If adding a file fails, print the exact command used for troubleshooting.
-
Cleanup or Error Handling
- If all files are added successfully, delete the work folder and print "Deployment Complete".
- If any file fails to add, do not delete the work folder and print "Deployment Failed" along with the error information and the failed command.
Additional Notes
- All paths are fully resolved and printed before any processing begins.
- Original file casing is preserved in the disk image.
- The final disk image will overwrite any existing file with the same name.
- Failures in file addition are clearly reported along with the command used.
Monday, February 17, 2025
## 3. How to Implement Amazon Rekognition for Your Web App
### Step 1: Set Up Amazon Rekognition on AWS
1. Sign up for AWS.
2. Create an IAM Role with Rekognition and S3 permissions.
3. Create a Face Collection (used for storing and matching faces).
4. Obtain API Credentials (Access Key & Secret Key).
Saturday, February 15, 2025
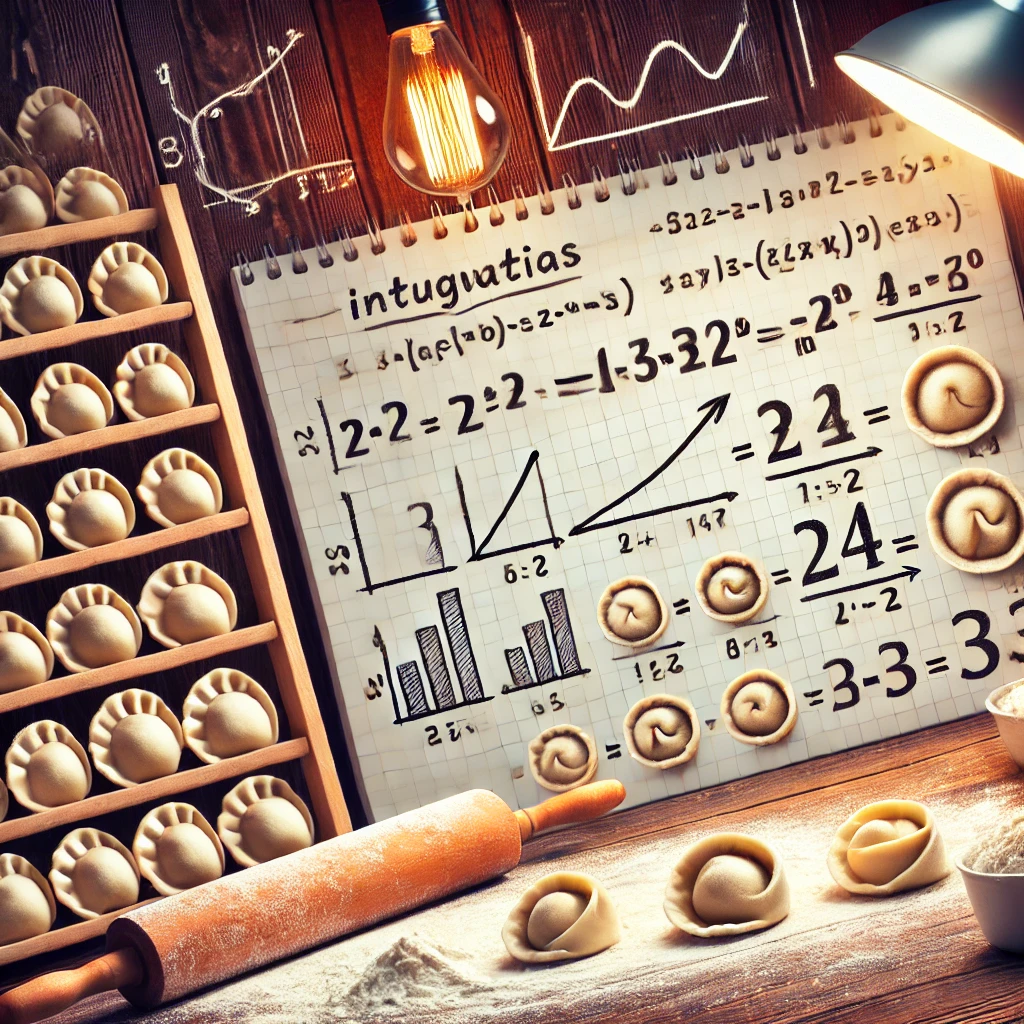
Π£ΠΌΠ΅Π½ΡΡΠ΅Π½ΠΈΠ΅ Π»ΠΈΠ½Π΅ΠΉΠ½ΠΎΠ³ΠΎ ΡΠ°Π·ΠΌΠ΅ΡΠ° ΠΏΠ΅Π»ΡΠΌΠ΅Π½Ρ Π² Π΄Π²Π° ΡΠ°Π·Π° ΠΏΡΠΈΠ²ΠΎΠ΄ΠΈΡ ΠΊ ΡΠΎΠΊΡΠ°ΡΠ΅Π½ΠΈΡ ΠΏΠ»ΠΎΡΠ°Π΄ΠΈ ΡΠ΅ΡΡΠ° Π² ΡΠ΅ΡΡΡΠ΅ ΡΠ°Π·Π°, Π° ΠΎΠ±ΡΠ΅ΠΌΠ° Π½Π°ΡΠΈΠ½ΠΊΠΈ β Π² Π²ΠΎΡΠ΅ΠΌΡ ΡΠ°Π·. ΠΡΠΎ ΡΠΎΠΎΡΠ½ΠΎΡΠ΅Π½ΠΈΠ΅ ΠΎΠ±ΡΡΡΠ½ΡΠ΅Ρ ΡΠΊΠΎΠ½ΠΎΠΌΠΈΡΠ΅ΡΠΊΡΡ Π²ΡΠ³ΠΎΠ΄Ρ Π΄Π»Ρ ΠΏΡΠΎΠΈΠ·Π²ΠΎΠ΄ΠΈΡΠ΅Π»Π΅ΠΉ, ΡΠ²ΡΠ·Π°Π½Π½ΡΡ Ρ ΡΠΌΠ΅Π½ΡΡΠ΅Π½ΠΈΠ΅ΠΌ Π΄ΠΎΠ»ΠΈ Π½Π°ΡΠΈΠ½ΠΊΠΈ ΠΏΡΠΈ ΡΠΎΡ
ΡΠ°Π½Π΅Π½ΠΈΠΈ ΠΎΠ±ΡΠ΅Π³ΠΎ ΠΊΠΎΠ»ΠΈΡΠ΅ΡΡΠ²Π° ΠΈΠ·Π΄Π΅Π»ΠΈΠΉ.
ΠΠ°Π½Π½ΡΠΉ ΡΡΡΠ΅ΠΊΡ ΠΏΠΎΠ΄ΡΠ²Π΅ΡΠΆΠ΄Π°Π΅ΡΡΡ ΠΌΠ°ΡΠ΅ΠΌΠ°ΡΠΈΡΠ΅ΡΠΊΠΈΠΌ ΡΠΎΠΎΡΠ½ΠΎΡΠ΅Π½ΠΈΠ΅ΠΌ: Π΄Π²ΠΎΠΉΠ½ΠΎΠΉ ΠΊΡΠΈΠ²ΠΎΠ»ΠΈΠ½Π΅ΠΉΠ½ΡΠΉ ΠΈΠ½ΡΠ΅Π³ΡΠ°Π» ΠΏΠΎ ΠΏΠΎΠ²Π΅ΡΡ
Π½ΠΎΡΡΠΈ ΡΠΊΠ²ΠΈΠ²Π°Π»Π΅Π½ΡΠ΅Π½ ΡΡΠΎΠΉΠ½ΠΎΠΌΡ ΠΈΠ½ΡΠ΅Π³ΡΠ°Π»Ρ ΠΏΠΎ ΠΎΠ±ΡΠ΅ΠΌΡ, ΠΎΠ³ΡΠ°Π½ΠΈΡΠ΅Π½Π½ΠΎΠΌΡ Π΄Π°Π½Π½ΠΎΠΉ ΠΏΠΎΠ²Π΅ΡΡ
Π½ΠΎΡΡΡΡ. Π ΡΠ΅Π·ΡΠ»ΡΡΠ°ΡΠ΅ ΡΠΌΠ΅Π½ΡΡΠ΅Π½ΠΈΠ΅ ΡΠ°Π·ΠΌΠ΅ΡΠ° ΠΏΠ΅Π»ΡΠΌΠ΅Π½Π΅ΠΉ Π²Π΅Π΄Π΅Ρ ΠΊ Π·Π½Π°ΡΠΈΡΠ΅Π»ΡΠ½ΠΎΠΌΡ ΡΠΎΠΊΡΠ°ΡΠ΅Π½ΠΈΡ ΠΎΠ±ΡΠ΅ΠΌΠ° Π½Π°ΡΠΈΠ½ΠΊΠΈ, ΡΡΠΎ ΠΌΠΎΠΆΠ΅Ρ ΠΈΡΠΏΠΎΠ»ΡΠ·ΠΎΠ²Π°ΡΡΡΡ ΠΊΠ°ΠΊ ΡΠΊΠΎΠ½ΠΎΠΌΠΈΡΠ΅ΡΠΊΠ°Ρ ΡΡΡΠ°ΡΠ΅Π³ΠΈΡ.
Π ΠΏΡΠΈΠΊΠ»Π°Π΄Π½ΠΎΠΌ Π°ΡΠΏΠ΅ΠΊΡΠ΅ Π΄Π°Π½Π½ΠΎΠ΅ ΡΠ²Π»Π΅Π½ΠΈΠ΅ ΠΌΠΎΠΆΠ΅Ρ ΠΈΠ½ΡΠ΅ΡΠΏΡΠ΅ΡΠΈΡΠΎΠ²Π°ΡΡΡΡ ΠΊΠ°ΠΊ ΡΡΡΠ°ΡΠ΅Π³ΠΈΡ ΡΠ½ΠΈΠΆΠ΅Π½ΠΈΡ ΡΠ΅Π±Π΅ΡΡΠΎΠΈΠΌΠΎΡΡΠΈ ΠΏΡΠΎΠΈΠ·Π²ΠΎΠ΄ΡΡΠ²Π° Π·Π° ΡΡΠ΅Ρ ΡΠΌΠ΅Π½ΡΡΠ΅Π½ΠΈΡ ΡΠΎΠ΄Π΅ΡΠΆΠ°Π½ΠΈΡ Π½Π°ΡΠΈΠ½ΠΊΠΈ ΠΏΡΠΈ ΡΠΎΡ
ΡΠ°Π½Π΅Π½ΠΈΠΈ ΠΎΠ±ΡΠ΅Π³ΠΎ ΠΊΠΎΠ»ΠΈΡΠ΅ΡΡΠ²Π° ΠΈΠ·Π΄Π΅Π»ΠΈΠΉ.
Tuesday, August 20, 2024
When handling CSV files in PHP, you have multiple options for reading the data, with fgetcsv() and the file() function being two common approaches. Each method has its advantages and disadvantages, depending on what you need to do with the CSV data. Letβs compare these two methods:
1. Using fgetcsv()
Advantages:
- Built for CSVs: fgetcsv() is specifically designed to read CSV files. It automatically handles CSV formatting, including encapsulated fields with commas, quotes, and escaped characters.
- Memory Efficiency: It reads one line at a time, which is memory efficient, especially useful for large files.
- Convenient Parsing: Automatically parses the line into an array based on the delimiter, which is very handy for direct data manipulation.
Disadvantages:
- Limited to CSV Format: Only useful for CSV files and cannot be easily used for other file formats.
- Less Flexible: You’re mostly stuck with the structure of how fgetcsv() parses each line (though you can specify custom delimiters, escape characters, and enclosures).
Example Usage of fgetcsv():
$handle = fopen('path/to/file.csv', 'r');
while (($data = fgetcsv($handle, 1000, ",")) !== false) {
print_r($data);
}
fclose($handle);
2. Using file()
Advantages:
- Simplicity: file() reads the entire file into an array, where each line is an element of the array, simplifying the process of file reading.
- Flexible Post-Processing: Useful if you need to process each line as a whole string or perform non-CSV specific parsing.
- Convenient for Smaller Files: Quickly reads smaller files into memory, making it easier to manipulate content that isnβt strictly structured as CSV.
Disadvantages:
- Memory Usage: Since it reads the entire file into memory at once, it can be inefficient or impractical for very large files.
- Manual Parsing Required: Does not handle CSV complexity such as commas in fields, quoted fields, etc., without additional parsing logic.
Example Usage of file():
$lines = file('path/to/file.csv');
foreach ($lines as $line) {
$data = str_getcsv($line); // Convert line to array similar to fgetcsv
print_r($data);
}
Decision Factors:
- File Size: Use fgetcsv() for large files to keep memory usage down. Use file() for smaller files where quick access to all lines is more convenient.
- Complexity of CSV: If the CSV data includes lots of special cases (like fields containing commas, quotes, or newlines), fgetcsv() handles these natively. If your data is simpler or requires custom processing, file() might be sufficient with manual parsing.
- Processing Needs: If you need to process or analyze each line of text beyond simple CSV parsing (like complex checks or multiple parsing passes), file() can be advantageous.
Friday, April 12, 2024
ΠΠ»Ρ ΡΠ΅Ρ
ΠΊΡΠΎ ΡΠΈΡΠ°Π΅Ρ ΡΡΠΎΡ ΠΏΠΎΡΡ Π½Π΅ 12 Π°ΠΏΡΠ΅Π»Ρ 2024 ΠΊΠΎΠ½ΡΠ΅ΠΊΡΡ: Π² ΡΠ΅Π·ΡΠ»ΡΡΠ°ΡΠ΅ ΠΈΠ·Π²Π΅ΡΡΠ½ΡΡ
ΡΠΎΠ±ΡΡΠΈΠΉ 7 ΠΎΠΊΡΡΠ±ΡΡ 2023Π³, ΠΏΠΎΡΠ»Π΅ 6 ΠΌΠ΅ΡΡΡΠ΅Π² Π²ΠΎΠΉΠ½Ρ, ΠΠ·ΡΠ°ΠΈΠ»Ρ ΡΠ΅ΡΠΈΠ» ΡΠ°Π·Π±ΠΎΠΌΠ±ΠΈΡΡ ΠΏΠΎΡΠΎΠ»ΡΡΡΠ²ΠΎ ΠΡΠ°Π½Π° Π½Π° ΡΠ΅ΡΡΠΈΡΠΎΡΠΈΠΈ Π‘ΠΈΡΠΈΠΈ. Π ΡΠ΅Π·ΡΠ»ΡΡΠ°ΡΠ΅ ΡΠΆΠ΅ Π½Π΅Π΄Π΅Π»Ρ Π²ΡΡ ΡΡΡΠ°Π½Π° ΡΠΈΠ΄ΠΈΡ Π½Π° ΠΈΠ·ΠΌΠ΅Π½Π΅ ΠΈ ΠΆΠ΄ΡΡ ΠΊΠΎΠ³Π΄Π° ΠΡΠ°Π½ Π΄ΠΎΠ»Π±Π°Π½ΡΡ Π² ΠΎΡΠ²Π΅Ρ.
ΠΠ·ΡΠ°ΠΈΠ»ΡΡΠΊΠ°Ρ ΠΏΡΠ΅ΡΡΠ°, Π° ΡΠΎΡΠ½Π΅Π΅ ΠΏΠΎΡΡΠ°Π» Π£Π°ΠΉΠΠ΅Ρ (Π±ΡΠ²ΡΠΈΠΉ Π―Π΄ΠΈΠΉΠΎΡ ΠΡ
Π°ΡΠΎΠ½ΠΎΡ), ΠΏΡΡΠ°ΡΡΡ ΡΡΠΏΠΎΠΊΠΎΠΈΡΡ Π½Π°ΡΠ΅Π»Π΅Π½ΠΈΠ΅, Π·Π°ΠΏΠΎΡΡΠΈΠ» ΠΊΠ°ΡΡΠΈΠ½ΠΊΡ ΡΠ»Π΅Π΄ΡΡΡΠ΅Π³ΠΎ ΡΠΎΠ΄Π΅ΡΠΆΠ°Π½ΠΈΡ: ΠΠ΅Π±ΠΎ Π·Π°ΠΊΡΡΡΠΎ! Π§Π΅ΡΡΡΠ΅ ΡΡΠΎΠ²Π½Ρ Π·Π°ΡΠΈΡΡ!
ΠΠ΅Π±ΠΎ Π·Π°ΠΊΡΡΡΠΎ! Π§Π΅ΡΡΡΠ΅ ΡΡΠΎΠ²Π½Ρ Π·Π°ΡΠΈΡΡ![/img]
Π ΡΠ²ΡΠ·ΠΈ Ρ ΡΡΠΈΠΌ, ΡΠ°ΡΡΠΊΠ°Π·…
ΠΠ°ΠΊ ΡΠΎ ΡΠ°Π· Π½Π°Ρ “ΠΏΠΎΠ»ΠΊ” ΠΠΠ ΡΠ΅ΡΠΈΠ» ΠΏΡΠΎΠ²Π΅ΡΡΠΈ ΡΡΠ΅Π½ΠΈΡ. Π‘ΠΎΠ±ΡΠ°Π»ΠΈ Π²ΡΠ΅Ρ
Π±ΠΎΠ»Π΅Π·Π½ΡΡ
, ΠΎΡΠΎΠ±ΡΠ°Π»ΠΈ Ρ Π½ΠΈΡ
“ΠΎΡΠ²ΠΎΠ±ΠΎΠΆΠ΄Π΅Π½ΠΈΡ ΠΎΡ ΡΠΈΠ·ΠΊΡΠ»ΡΡΡΡΡ”, ΠΏΠΎΠ³ΡΡΠ·ΠΈΠ»ΠΈ Π² Π³ΡΡΠ·ΠΎΠ²ΠΈΠΊΠΈ ΠΈ ΠΎΡΠΏΡΠ°Π²ΠΈΠ»ΠΈ ΡΡΠ΄Π° ΠΊΡΠ΄Π° Ρ
ΡΡΠΈΡΡ Π½Π΅ ΡΡΡΠ΅Π»ΡΠ»ΠΈ, ΡΠΎ Π΅ΡΡΡ ΡΡΠ΄Π° Π³Π΄Π΅ Π½Π°ΠΏΠΈΡΠ°Π½ΠΎ “ΠΊΠΎΠ½Π΅Ρ ΡΠ²Π΅ΡΠ°” ΠΈ ΠΎΡΡΡΠ΄Π° Π΅ΡΠ΅ 15ΠΊΠΌ Π½Π°Π»Π΅Π²ΠΎ.
ΠΠΎ ΠΏΡΠΈΠ±ΡΡΠΈΠΈ ΡΠ°Π·Π²Π΅ΡΠ½ΡΠ»ΠΈ ΠΎΠ½ΠΈ ΡΠ°ΠΌ ΡΠ²ΠΎΠΈ Π·Π°ΠΌΠ΅ΡΠ°ΡΠ΅Π»ΡΠ½ΡΠ΅ Π°ΠΌΠ΅ΡΠΈΠΊΠ°Π½ΡΠΊΠΈΠ΅ Π²ΠΎΠΎΡΡΠΆΠ΅Π½ΠΈΡ, ΠΊΠΎΡΠΎΡΡΠ΅ ΡΠΆΠ΅ ΡΠΎΠ³Π΄Π° ΠΏΠΎΡΠ° Π±ΡΠ»ΠΎ ΠΎΡΠΏΡΠ°Π²ΠΈΡΡ Π²ΠΎ ΠΡΠ΅ΡΠ½Π°ΠΌ Π½Π° Π²ΡΡΡΠ°Π²ΠΊΡ “Π‘ ΠΏΠΎΠΌΠΎΡΡΡ ΡΡΠΎΠ³ΠΎ ΠΈ Π‘Π‘Π‘Π ΠΌΡ ΠΏΠΎΠ±Π΅Π΄ΠΈΠ»ΠΈ Π‘Π¨Π 25 Π»Π΅Ρ Π½Π°Π·Π°Π΄”. ΠΠ°ΡΡΠ½ΡΠ»ΠΈ ΡΠ΅Π½Ρ, Π΄ΠΎΡΡΠ°Π»ΠΈ ΠΏΠΈΠ²ΠΎ ΠΈ ΡΠ΅Π»ΠΈ ΠΆΠ΄Π°ΡΡ ΠΊΠΎΠ³Π΄Π° ΡΠ°Π΄Π°Ρ ΠΏΠΎΠ΄Π°ΡΡ Π·Π²ΡΠΊΠΎΠ²ΠΎΠΉ ΡΠΈΠ³Π½Π°Π» ΠΎΠ± ΠΎΠ±Π½Π°ΡΡΠΆΠ΅Π½ΠΈΠΈ ΡΡΠ΅Π±Π½ΠΎΠΉ ΡΠ΅Π»ΠΈ.
Π‘ΠΈΠ³Π½Π°Π» Π½Π΅ Π·Π°ΡΡΠ°Π²ΠΈΠ» ΡΠ΅Π±Ρ ΠΆΠ΄Π°ΡΡ! Π’ΠΎΠ»ΡΠΊΠΎ ΠΈΡΡ
ΠΎΠ΄ΠΈΠ» ΠΎΠ½ Π½Π΅ ΠΎΡ ΡΠ°Π΄Π°ΡΠ½ΠΎΠΉ ΡΡΡΠ°Π½ΠΎΠ²ΠΊΠΈ, Π° ΠΎΡ ΡΠ΅Π»Π΅ΡΠΎΠ½Π° Π½Π° ΠΊΠΎΠΎΡΠ΄ΠΈΠ½ΡΠΈΠΎΠ½Π½ΠΎΠΌ ΠΏΡΠ½ΠΊΡΠ΅, Π·Π° 70ΠΊΠΌ ΠΎΡ ΠΏΡΡΠΊΠΎΠ²ΠΎΠΉ ΡΡΡΠ°Π½ΠΎΠ²ΠΊΠΈ. Π£ΠΆΠ΅ ΡΠΎΠ³Π΄Π° Ρ Π½Π°Ρ Π·Π½Π°Π»ΠΈ ΡΡΠΎ ΡΠ°ΠΊΠΎΠ΅ ΡΠ°Π±ΠΎΡΠ° Π½Π° ΡΠ΄Π°Π»ΡΠ½ΠΊΠ΅. Π€ΠΎΡΠΌΠ°Ρ ΡΠΈΠ³Π½Π°Π»Π° Π±ΡΠ» ΡΠ°ΠΊΠΎΠΉ: Π·Π²ΠΎΠ½ΠΎΠΊ-Π·Π²ΠΎΠ½ΠΎΠΊ-ΡΡΠ΄ΠΎΠ²ΠΎΠΉ Π Π°Π±ΠΈΠ½ΠΎΠ²ΠΈΡ ΡΠ»ΡΡ
Π°Ρ!
ΠΠ°ΠΊ Π²Ρ Π½Π°Π²Π΅ΡΠ½ΠΎΠ΅ Π΄ΠΎΠ³Π°Π΄Π°Π»ΠΈΡΡ (Π·Π½Π°Ρ ΡΡΠΎ Π½Π΅Ρ) Π·Π²ΠΎΠ½ΠΈΠ» ΠΊΠΎΠΌΠ°Π½Π΄ΡΡΡΠΈΠΉ ΠΠΠ ΠΈ ΡΠΏΡΠ°ΡΠΈΠ²Π°Π», ΡΠ΄Π°Π»ΠΎΡΡ Π»ΠΈ Π½Π°ΠΌ Π·Π°ΡΠ΅ΡΡ ΡΠ΅Π»Ρ ΠΈ ΠΏΡΠΎΠ²Π΅ΡΡΠΈ “ΠΏΡΡΠΊ”, ΠΏΠΎΡΠΎΠΌΡ ΡΡΠΎ ΠΏΠΈΠ»ΠΎΡ ΡΡΠ΅Π±Π½ΠΎΠΉ ΡΠ΅Π»ΠΈ ΡΠΆΠ΅ ΠΏΠΎΠΎΠ±Π΅Π΄Π°Π», Π΄ΠΎΠΏΠΈΠ» ΠΊΠΎΠΌΠΏΠΎΡ ΠΈ Ρ
ΠΎΡΠ΅Ρ Π΅Ρ
Π°ΡΡ Π΄ΠΎΠΌΠΎΠΉ ΠΊ ΠΌΠ°ΠΌΠ΅.
Π ΠΎΠ±ΡΠ΅ΠΌ ΠΏΠΈΠ»ΠΎΡ Π½Π°ΡΠ΅Π·Π°Π» Π΅ΡΠ΅ 3 ΠΊΡΡΠ³Π° ΠΈ ΠΊ Π΄ΠΎΠΌΠΎΠΉ Π½Π΅ ΠΏΠΎΠ΅Ρ
Π°Π», ΠΏΠΎΡΠΎΠΌΡ ΠΊΠ°ΠΊ Π¨Π°Π±Π°Ρ ΠΈ Π°Π²ΡΠΎΠ±ΡΡΠΎΠ² Π±ΠΎΠ»ΡΡΠ΅ Π½Π΅Ρ.
ΠΠ°ΠΌΠ΅Π½ΡΡ ΠΏΠΈΡΠ°ΡΡ Π·Π΄Π΅ΡΡ